Object type refers to the structure of an object.
Infered Structure
Here is a JavaScript object literal:
const person = {
name: "Joby",
age: 37,
};
From the initialized value, TypeScript infers the type as:
const person: {
name: string;
age: number;
};
Now, if we try to create a new property, TypeScript throws an error.
person.country = "USA"; // Error
Generic Object Type
While assigning the object, we can explicitly provide an object
type.
const person: object = {
name: "Joby",
age: 37,
};
But Generic object
type is not much of use. We normally want the object structure to be well defined. Generic object type helps us to handle situations where we do not know the structure of the object before hand.
Using generic object type also does not perform well with intellisense.
Object Structure
We can also provide the object type as shown below:
const person: {
name: string;
age: number;
} = {
name: "Joby",
age: 37,
};
This is explicit type declaration. This performs well in intellisense.
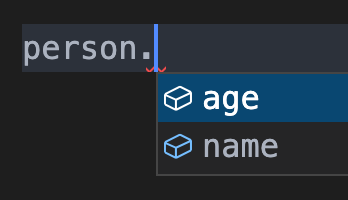